通过覆盖 fields() 和 extraFields() 方法,可以定义一些数据放入回应中。这两种方法之间的差别在于,前者定义了默认设置的字段,包函在响应中;而后者如果最终用户的请求通过扩展查询参数,之后可以在响应包函附加字段。
第1步 - 修改 models/MyUser.php 并使用以下代码:
<?php namespace app\models; use app\components\UppercaseBehavior; use Yii; /** * This is the model class for table "user". *@property integer $id * @property string $name * @property string $email */ class MyUser extends \yii\db\ActiveRecord { public function fields() { return [ 'id', 'name', //PHP callback 'datetime' => function($model) { return date("Y-m-d H:i:s"); } ]; } /** * @inheritdoc */ public static function tableName() { return 'user'; } /** * @inheritdoc */ public function rules() { return [ [['name', 'email'], 'string', 'max' => 255] ]; } /** * @inheritdoc */ public function attributeLabels() { return [ 'id' => 'ID', 'name' => 'Name', 'email' => 'Email', ]; } } ?>
除了默认的字段:id 和 name,这增加了一个自定义字段 - datetime。
第2步 - 在 Postman,运行通过URL :http://localhost:8080/users
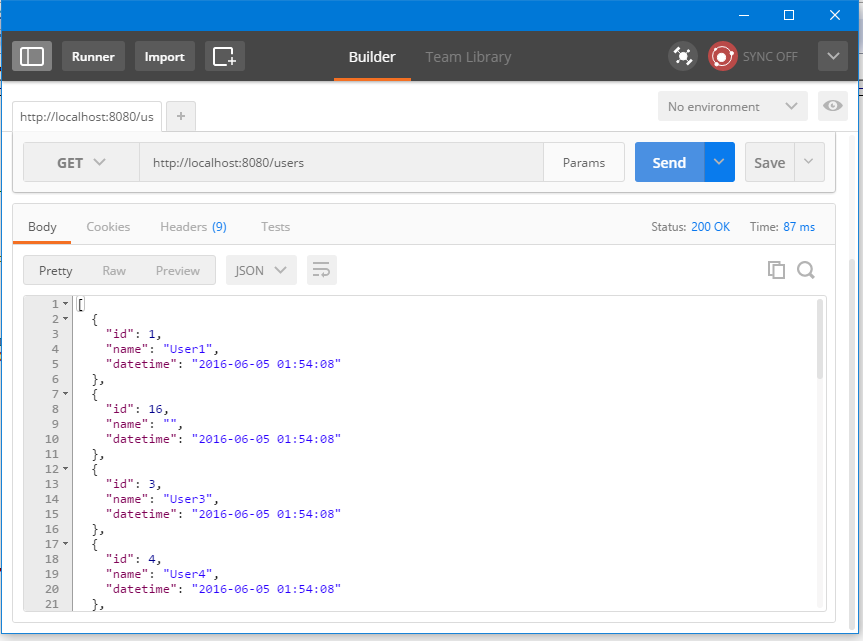
第3步 - 现在,修改用户模型:models/MyUser.php 并使用以下代码:
<?php namespace app\models; use app\components\UppercaseBehavior; use Yii; /** * This is the model class for table "user". * * @property integer $id * @property string $name * @property string $email */ class MyUser extends \yii\db\ActiveRecord { public function fields() { return [ 'id', 'name', ]; } public function extraFields() { return ['email']; } /** * @inheritdoc */ public static function tableName() { return 'user'; } /** * @inheritdoc */ public function rules() { return [ [['name', 'email'], 'string', 'max' => 255] ]; } /** * @inheritdoc */ public function attributeLabels() { return [ 'id' => 'ID', 'name' => 'Name', 'email' => 'Email', ]; } } ?>
请注意,email 字段是由 extraFields()方法返回。
第4步 - 要获取此字段的数据,运行:http://localhost:8080/users?expand=email 如下结果:
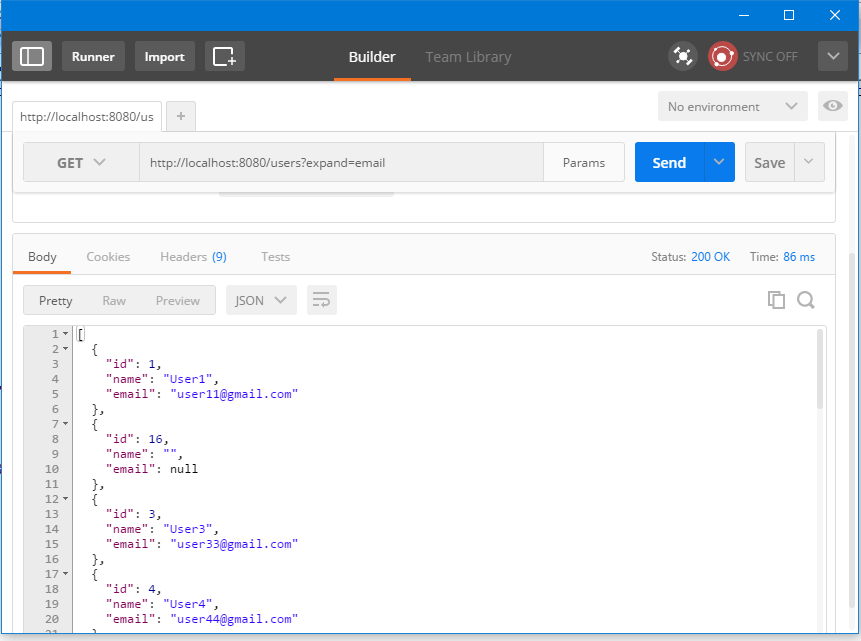
自定义操作
yii\rest\ActiveController 类提供了以下操作(动作) -
-
Index − 逐页列出资源
-
View − 返回指定资源的详细信息
-
Create − 创建一个新的资源
-
Update − 更新一个已存在的资源
-
Delete − 删除指定的资源
-
Options − 返回支持的HTTP方法
以上所有动作在 method() 方法中声明。
要禁用 “delete” 和 “create” 的动作,修改 UserController.php 并使用以下代码 -
<?php namespace app\controllers; use yii\rest\ActiveController; class UserController extends ActiveController { public $modelClass = 'app\models\MyUser'; public function actions() { $actions = parent::actions(); // disable the "delete" and "create" actions unset($actions['delete'], $actions['create']); return $actions; } } ?>
错误处理
当获得一个RESTful API请求,如果该请求错误或出现一些意想不到的东西在服务器上发生,可以简单地抛出一个异常。
如果能找出错误的原因,应该使用一个适当的 HTTP 状态码来抛出异常。
Yii REST 使用以下状态 -
-
200 − OK/正常完成
-
201 − 一个资源成功创建用来响应POST请求。 Location头包含一个指向新创建资源的URL。
-
204 − 请求被成功处理并响应但不包含内容
-
304 − 资源没有被修改
-
400 − 错误的请求
-
401 − 验证失败
-
403 − 通过身份验证的用户不允许访问指定的API端点
-
404 − 资源不存在
-
405 − 不被允许的方法
-
415 − 不支持的媒体类型
-
422 − 数据验证失败
-
429 − 过多的请求
-
500 − 内部服务器错误
上一篇:
Yii动作使用RESTful API
下一篇:
Yii测试